This example was ported from the PyQt4 version by Gu冒j贸n Gu冒j贸nsson.
Introduction
I have some difficulties to find an appropriate way to solve a problem of communication between objects in differents threads (although I already read the Signals/slots accross threads). Here is my problem: I have an object a of class A living.
Wynn las vegas to lucky dragon casino. Nightlife: Duelling Piano Bars/ Lounges.
In some applications it is often necessary to perform long-running tasks, such as computations or network operations, that cannot be broken up into smaller pieces and processed alongside normal application events. In such cases, we would like to be able to perform these tasks in a way that does not interfere with the normal running of the application, and ensure that the user interface continues to be updated. One way of achieving this is to perform these tasks in a separate thread to the main user interface thread, and only interact with it when we have results we need to display.
This example shows how to create a separate thread to perform a task - in this case, drawing stars for a picture - while continuing to run the main user interface thread. The worker thread draws each star onto its own individual image, and it passes each image back to the example's window which resides in the main application thread.
The User Interface
We begin by importing the modules we require. We need the math and random modules to help us draw stars.
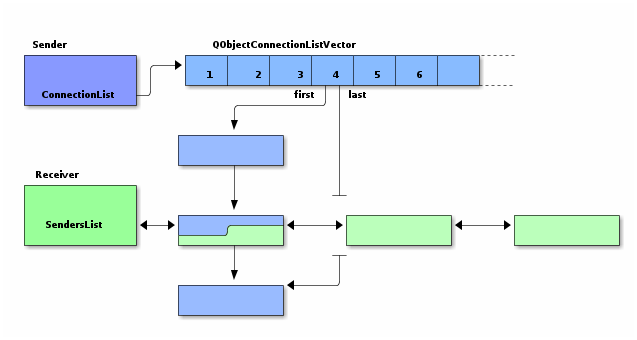
The main window in this example is just a QWidget. We create a single Worker instance that we can reuse as required.
Signal And Slot In Qt
The user interface consists of a label, spin box and a push button that the user interacts with to configure the number of stars that the thread wil draw. The output from the thread is presented in a QLabel instance, viewer.
We connect the standard finished() and terminated() signals from the thread to the same slot in the widget. This will reset the user interface when the thread stops running. The custom output(QRect, QImage) signal is connected to the addImage() slot so that we can update the viewer label every time a new star is drawn.
The start button's clicked() signal is connected to the makePicture() slot, which is responsible for starting the worker thread.
We place each of the widgets into a grid layout and set the window's title:
The makePicture() slot needs to do three things: disable the user interface widgets that are used to start a thread, clear the viewer label with a new pixmap, and start the thread with the appropriate parameters.
Since the start button is the only widget that can cause this slot to be invoked, we simply disable it before starting the thread, avoiding problems with re-entrancy.
We call a custom method in the Worker thread instance with the size of the viewer label and the number of stars, obtained from the spin box.
Whenever is star is drawn by the worker thread, it will emit a signal that is connected to the addImage() slot. This slot is called with a QRect value, indicating where the star should be placed in the pixmap held by the viewer label, and an image of the star itself:
We use a QPainter to draw the image at the appropriate place on the label's pixmap.
The updateUi() slot is called when a thread stops running. Since we usually want to let the user run the thread again, we reset the user interface to enable the start button to be pressed:

Now that we have seen how an instance of the Window class uses the worker thread, let us take a look at the thread's implementation.
The Worker Thread
The worker thread is implemented as a PyQt thread rather than a Python thread since we want to take advantage of the signals and slots mechanism to communicate with the main application.
We define size and stars attributes that store information about the work the thread is required to do, and we assign default values to them. The exiting attribute is used to tell the thread to stop processing.
Each star is drawn using a QPainterPath that we define in advance:
Before a Worker object is destroyed, we need to ensure that it stops processing. For this reason, we implement the following method in a way that indicates to the part of the object that performs the processing that it must stop, and waits until it does so.
For convenience, we define a method to set up the attributes required by the thread before starting it.
The start() method is a special method that sets up the thread and calls our implementation of the run() method. We provide the render() method instead of letting our own run() method take extra arguments because the run() method is called by PyQt itself with no arguments.
The run() method is where we perform the processing that occurs in the thread provided by the Worker instance:
Information stored as attributes in the instance determines the number of stars to be drawn and the area over which they will be distributed.
We draw the number of stars requested as long as the exiting attribute remains False. This additional check allows us to terminate the thread on demand by setting the exiting attribute to True at any time.
The drawing code is not particularly relevant to this example. We simply draw on an appropriately-sized transparent image.
For each star drawn, we send the main thread information about where it should be placed along with the star's image by emitting our custom output() signal:
Since QRect and QImage objects can be serialized for transmission via the signals and slots mechanism, they can be sent between threads in this way, making it convenient to use threads in a wide range of situations where built-in types are used.
Running the Example
We only need one more piece of code to complete the example:
Qt does not provide support for using the Signals & Slots mechanism in combination with C++ templates. See: https://doc.qt.io/qt-5/why-moc.html
However: It is actually possible to do this, when one is willing to keep track of the things normally handledby the QObject::connect() function and 'call' slots with QMetaObject::invokeMethod().
One of the major benefits of playing at on online Poker site should you live or be visiting any part of the US State of Washington, is that there are some excellent promotional offers and very high paying Poker Tournaments always on offer which will give you the maximum winning chances and the maximum winning potential. Play poker online washington state. Jul 17, 2018 In January 2015, HB 1114 was introduced, a bill to legalize poker online in Washington, by state Representative Sherry Appleton. The following month the state announced the bill would not be getting a hearing this session due to a lack of support. Playing Online Poker in the State of Washington - How to Get Away w/ It Washington is a very interesting state when it comes to talking about online poker. While they have laws on the books that can make playing poker up to a Class C felony, the state has NEVER enforced this law.
Online casino with fast payouts. Coming in our trifecta of where to play your game at the best online casino for real money in the USA is Slots.lv. Slots.lv is where you go to play and win real money online casino. Slots.lv is the slot players paradise. With all the slot games created, slots.lv offers an incredible $5000 deposit incentive! The USA's fastest paying online casino sites offer the best in all departments including: top quality customer service, high percentage odds, fast deposit and withdrawals, even instant payouts.
Doing this has some nice side effects like not having to call qRegisterMetaType() or using the Q_DECLARE_METATYPE macro.
For a more detailed explanation take a look at the documentation of the invokeInContext() function in Magic.h
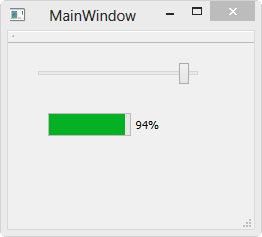
Furthermore does Qt provide mechanisms for threading, as being described here: https://doc.qt.io/qt-5/threads-technologies.html#choosing-an-appropriate-approach
The case 'Have an object living in another thread that can perform different tasks upon request and/or can receive new data to work with'
has the proposed solution 'Subclass a QObject to create a worker. Instantiate this worker object and a QThread. Move the worker to the new thread. Send commands or data to the worker object over queued signal-slot connections'
which implies not being able to use C++ templates, when relying solely on the native Signals & Slots mechanism.
But since I am lazy and don't like Copy & Paste, this framework provides a solution for handling that case in combination with C++ templates.
The main idea is to have 'Tasks' and 'Results', which are being described by template parameters 'T' and 'R' in this framework.
A Worker, which is running in his own thread, receives a task and responds with a result.
In general do you need to inherit from the Processor template class, the Worker template classand implement at least the pure virtual methods to create the functionality you want and give instances of thosenew classes to a Controller. That's it.
In order to be able to start working on new tasks, change the number of threads to use etc. you need a communication channelto the instance of the Processor.
You may use Qt Signals & Slots or use the invokeInContext() function Signals & Slots mechanism of this framework.The first example in src/examples/one/
covers all of that.
The second example in src/examples/two/
focuses only on the Signals & Slots system of this framework.
A third example in src/examples/three/
shows usage of the threading architecture + Signals and Slots of this frameworkcompletely without using Qt Signals and Slots.
Qt Signal Slot Thread
Everything you need is documented in the CMakeLists.txt
.
If you don't know what to do with such a file, you should use a search engine to find out.
Qt Connect Signal Slot
Use the Doxyfile
to generate the documentation via Doxygen
.
Qt Signals And Slots Tutorial
Look into the src/examples/
folder.